Hello, everybody. This is my first post. In this article, I'll demonstrate how to use Python and the Selenium library to open a browser and login to an account.
First, we'll need the following:
1. Run pip install selenium to install the Selenium library.
2. Install IEDriverServer.exe, it is a program that allows you to connect to the Internet Explorer driver server. In this case, I'm using Internet Explorer, so I install it here.
3. Install Java Runtime Environment,
make sure to choose the correct version for your computer.
● This is the login interface:
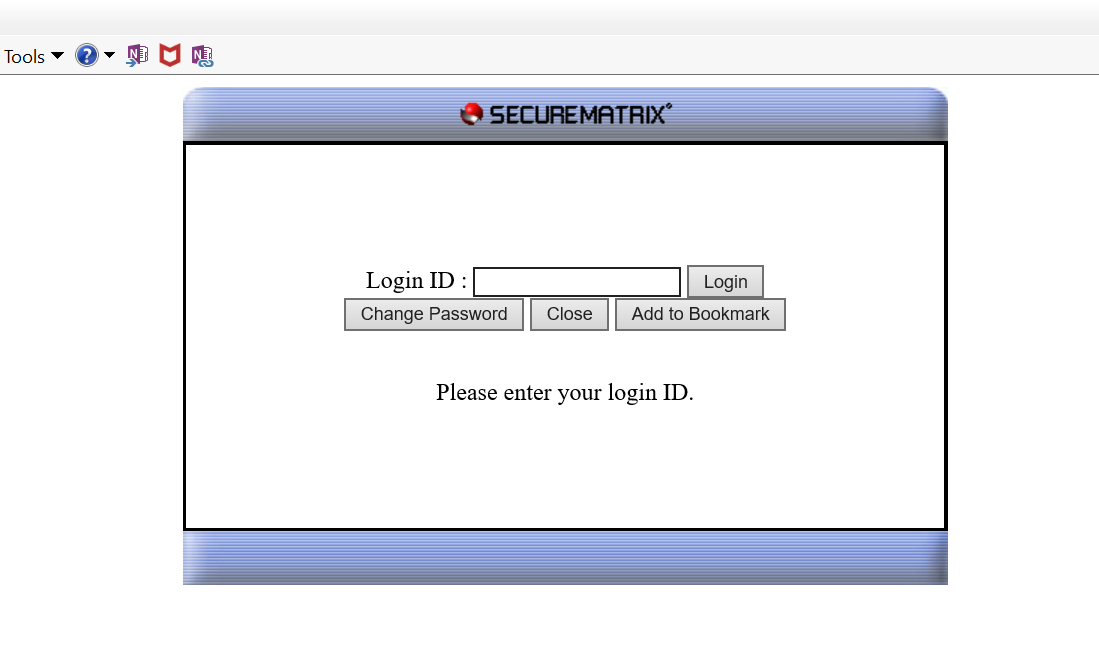
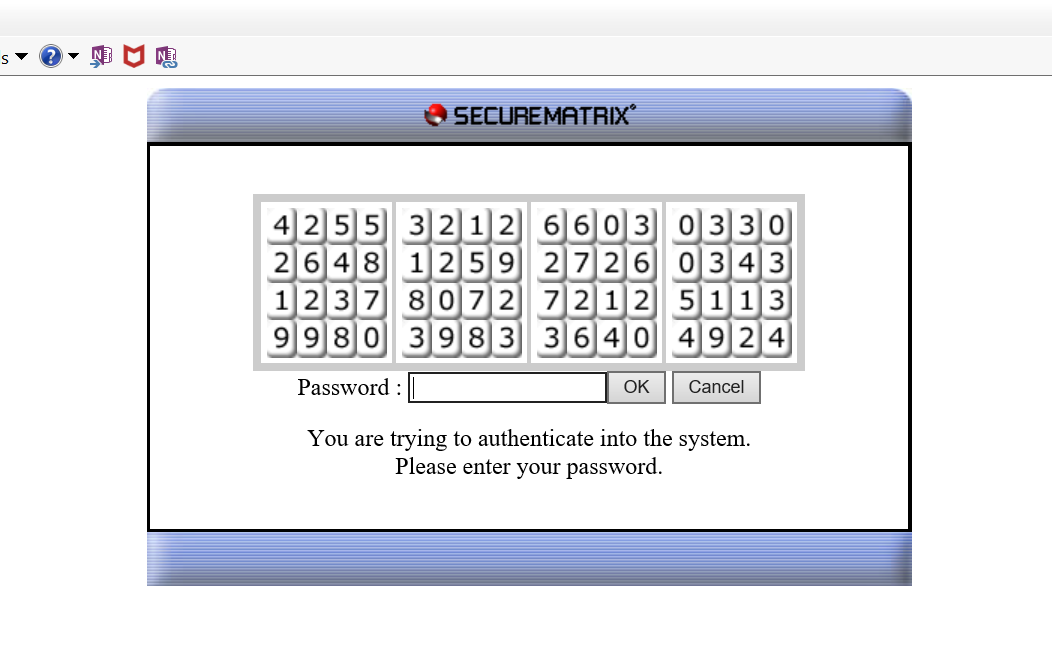
● SECUREMATRIX is a unique and highly secure.
Each time you log in, the number is changed, so the password will change also. Therefore, I make this tool to login automatically regardless of changed number.
● For example, my password will be 42199803 as shown below.
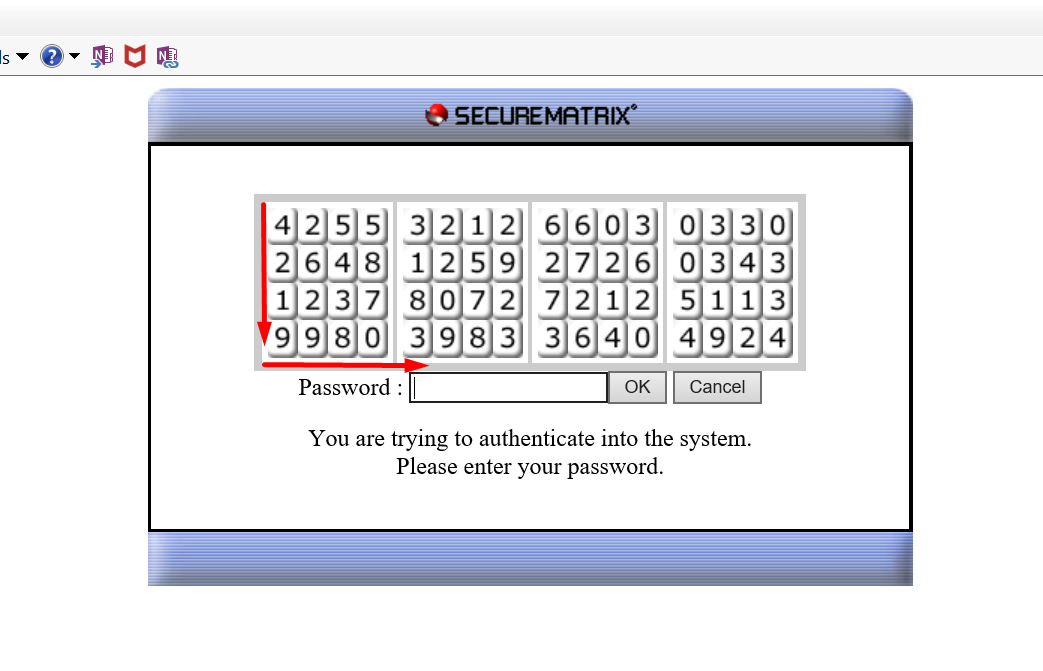
● We will press F12 to find elements of these numbers. The first number is "4" (attribute "alt"="4") so it will be "SMX_BTN_0".
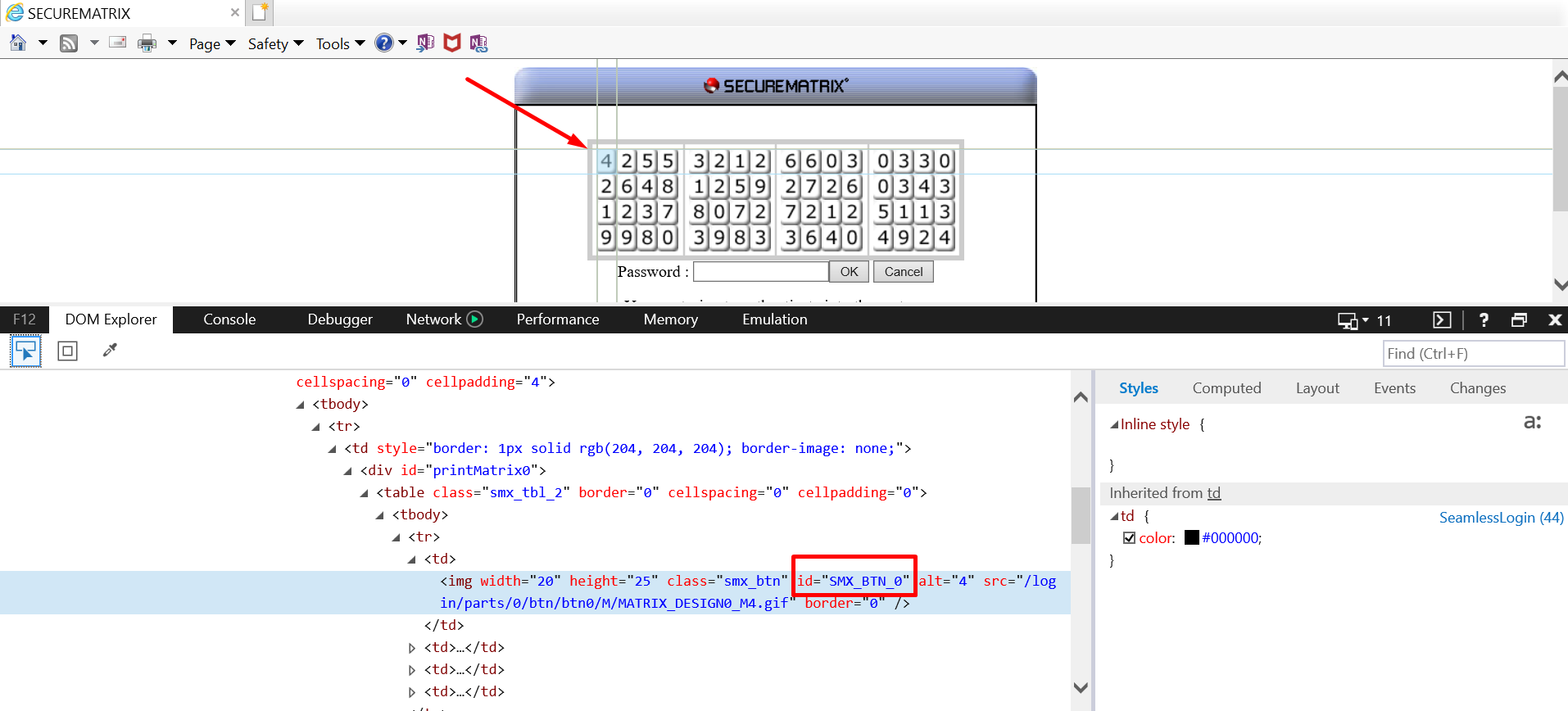
● We have a maxtrix table ID as blelow:

● Likewise, the IDs of the numbers in my password would be "SMX_BTN_0", "SMX_BTN_4", "SMX_BTN_8", "SMX_BTN_12", "SMX_BTN_13", "SMX_BTN_14", "SMX_BTN_15", "SMX_BTN_28".
Steps to do:
● We need install Selenium library first.
1 2 3 4 5 6 | #Library from selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC from time import sleep |
● Then, we will modify the code to apply for our account. We will edit user name, the path of IE driver and the link of website (line 2, 5 and 8).
1 2 3 4 5 6 7 8 9 | #User name userName='a512xxxx' #Path of IE Driver driver = webdriver.Ie("D:\Downloads\IEDriverServer.exe") #Link login driver.get("https://b2b-gateway.(hidden_name).com/login/ssl") driver.implicitly_wait(15) |
● We will find the elements of website. As the following img, the box is used to fill the user name would be "LOGIN_ID". Then we will send the value of user name to it and find the button "Login" (class name is "btnCustom") and click it.
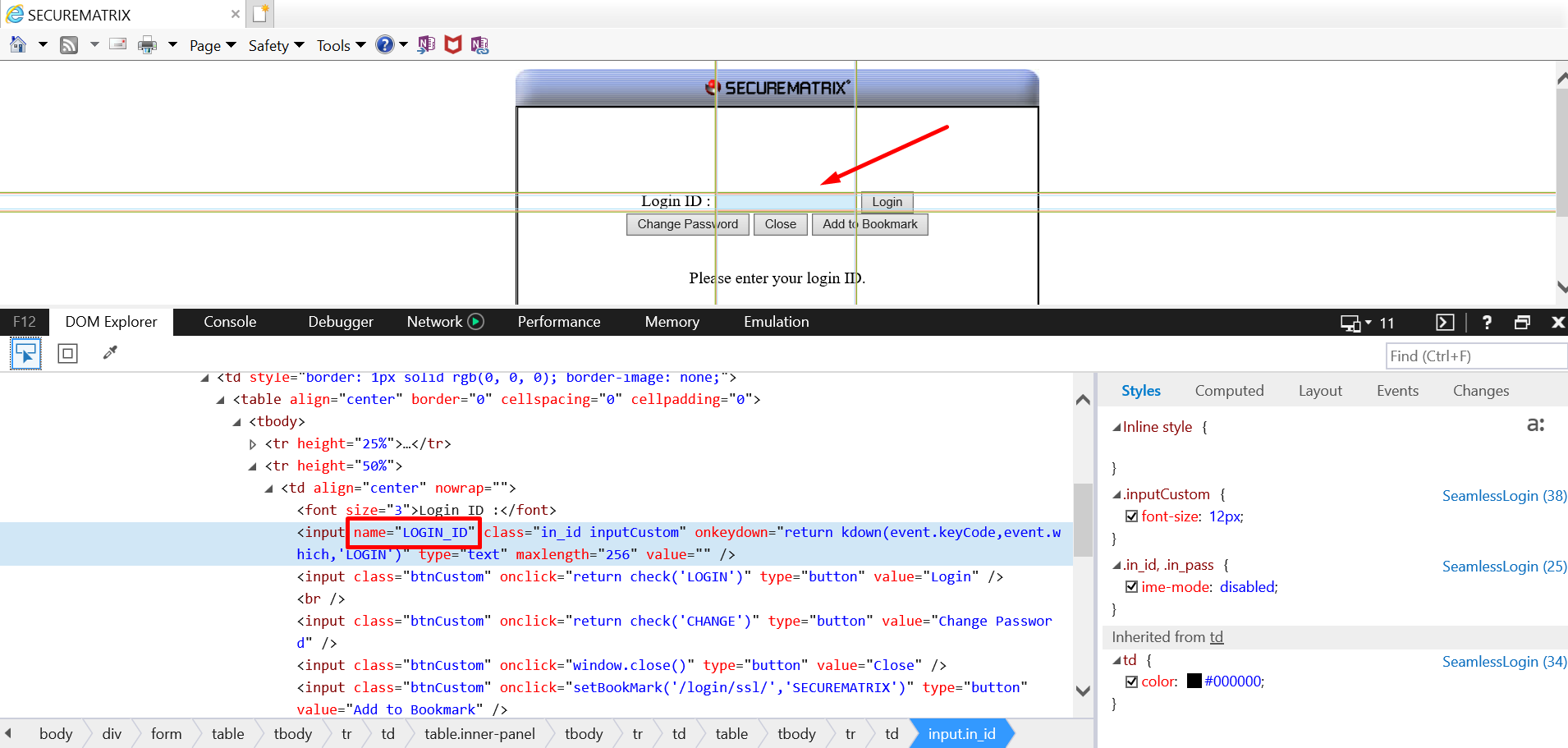
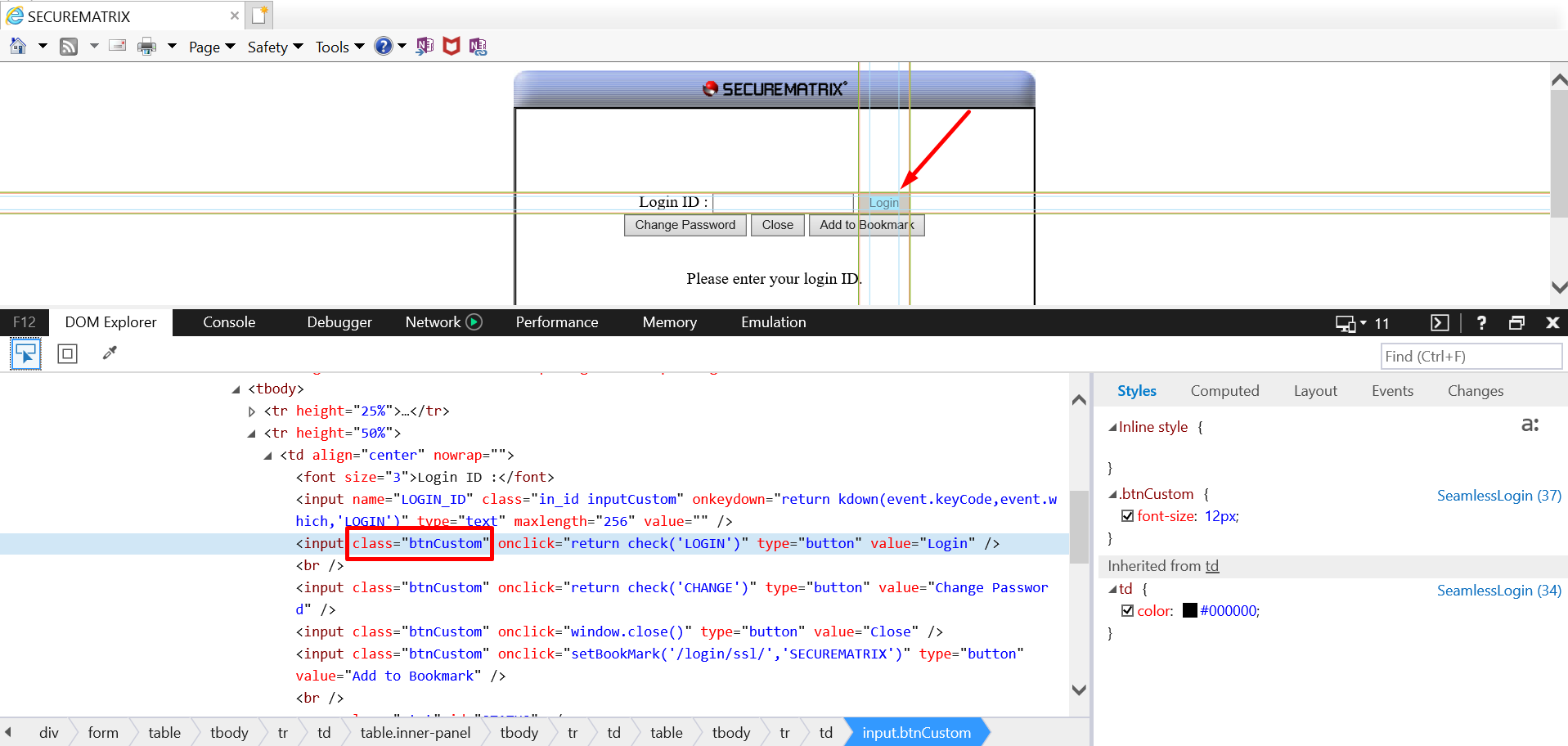
1 2 3 | #Find element and send data driver.find_element(By.NAME,'LOGIN_ID').send_keys(userName) driver.find_element(By.CLASS_NAME,'btnCustom').click() |
● Next, I will use the ID password I have found in above steps and put it in a for loop. Same as above, we will find the box to fill password and click the button.
1 2 3 4 5 6 7 8 9 10 | #Initialize password and fill password password="" arr = ["SMX_BTN_0", "SMX_BTN_4", "SMX_BTN_8", "SMX_BTN_12", "SMX_BTN_13", "SMX_BTN_14", "SMX_BTN_15", "SMX_BTN_28"] for i in arr: passTemp = driver.find_element(By.ID,i).get_attribute("alt") password += passTemp driver.find_element_by_name("PASSWORD").send_keys(password) button = driver.find_element_by_class_name("btnCustom").click() print("Waiting...") |
● Finally, I use "try-except" to check if there are any errors and inform to user via console output.
1 2 3 4 5 6 7 8 9 | #Check if there are any errors try: element = WebDriverWait(driver, 100).until(EC.presence_of_element_located((By.ID, "table_welcome_2"))) except: print("Could not connect to B2b. Please try again") finally: print("Welcome to the Secure Access SSL VPN, " + driver.find_element_by_class_name("cssSmall").text) print("Quit IE.") driver.quit() |
Here is full of source code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | #Library from selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC from time import sleep #User name userName='a512xxxx' #Path of IE Driver driver = webdriver.Ie("D:\Downloads\IEDriverServer.exe") #Link login driver.get("https://b2b-gateway.(hidden_name).com/login/ssl") driver.implicitly_wait(15) #Find element and send data driver.find_element(By.NAME,'LOGIN_ID').send_keys(userName) driver.find_element(By.CLASS_NAME,'btnCustom').click() #Initialize password and fill password password="" arr = ["SMX_BTN_0", "SMX_BTN_4", "SMX_BTN_8", "SMX_BTN_12", "SMX_BTN_13", "SMX_BTN_14", "SMX_BTN_15", "SMX_BTN_28"] for i in arr: assTemp = driver.find_element(By.ID,i).get_attribute("alt") password += passTemp driver.find_element_by_name("PASSWORD").send_keys(password) button = driver.find_element_by_class_name("btnCustom").click() print("Waiting...") #Check if there are any errors try: element = WebDriverWait(driver, 100).until(EC.presence_of_element_located((By.ID, "table_welcome_2"))) except: print("Could not connect to B2b. Please try again") finally: print("Welcome to the Secure Access SSL VPN, " + driver.find_element_by_class_name("cssSmall").text) print("Quit IE.") driver.quit() |