In this article, I'll demonstrate how to use Redmine library and Python to get my task to create weekly report on Confluence.
I will get name of the task via the time I have logged.
First, I have to create a datepicker to choose the date in a week.
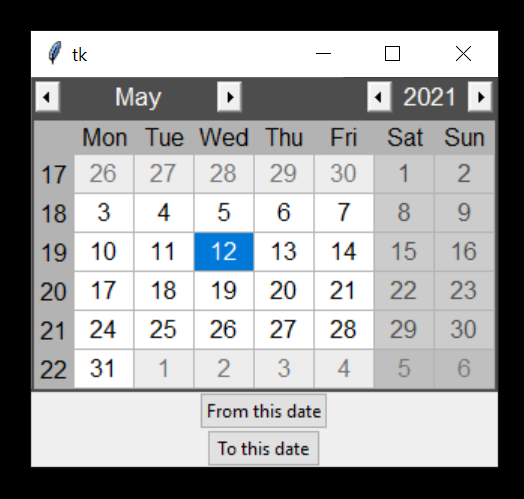
Second, I have to log in to Redmine address by Python. There are 2 ways to log in, they are:
1. Log in via user name and password.
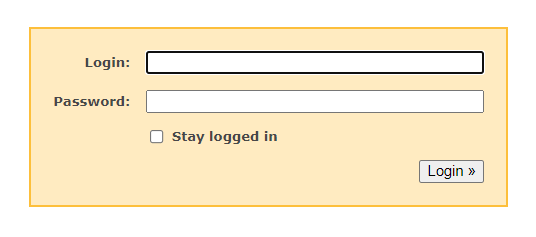
2. Log in via API access key: From home screen, go My account -> API access key.
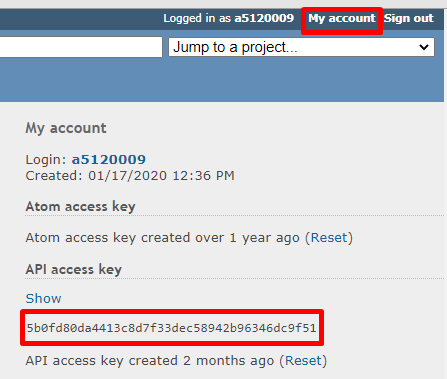
In this case, I used method 2.
Steps to do:
● We need install library first:
1. Run pip install python-redmine to install the Redmine library. Python-Redmine is a library for communicating with a Redmine project management application.
2. Run pip install tkcalendar to install the tkcalendar library. tkcalendar is a python module that provides the Calendar and DateEntry widgets for Tkinter.
1 2 3 4 | import tkinter as tk from tkinter import ttk from tkcalendar import Calendar, DateEntry from redminelib import Redmine |
● Next, we will modify correct the information accordingly.
1. API access key as above.
2. User ID.
User ID will will display in the corner of screen when moving the mouse over your login ID. My user ID is 5158.
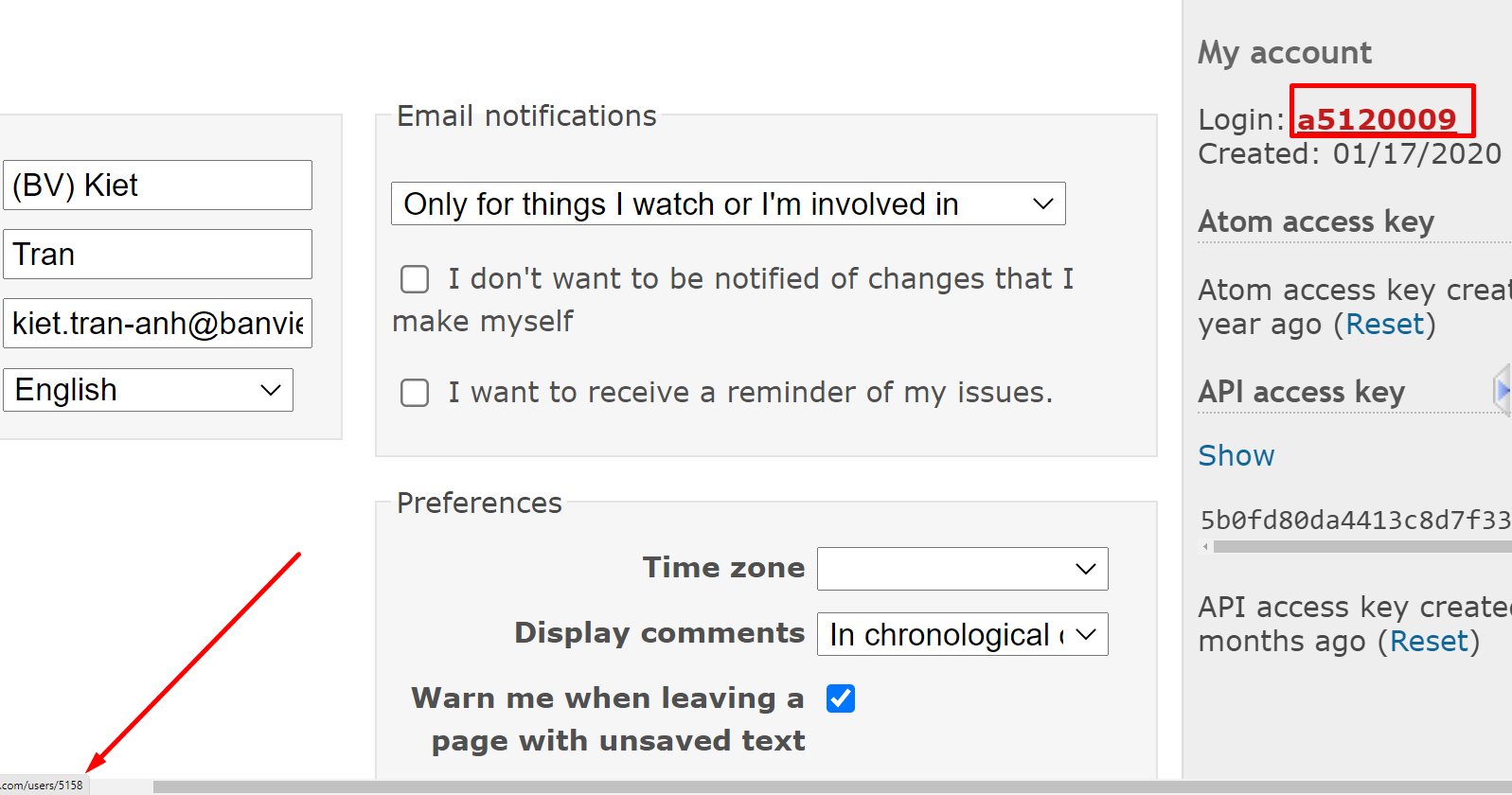
1 2 3 | # ------- Modify here ------- # keyRedmine="5b0fd80da4413c8d7f33dec58942b96346dc9f51" userID="5158" |
● We will create a date picker widget from tkcalendar.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | root = tk.Tk() def Get_from_date(): global fromDate fromDate = str(cal.selection_get()) def Get_to_date(): global toDate toDate = str(cal.selection_get()) cal = Calendar(root,font="Arial 12", selectmode='day',cursor="hand2") cal.pack(fill="both", expand=True) ttk.Button(root, text="From this date", command=Get_from_date).pack() ttk.Button(root, text="To this date", command=Get_to_date).pack() root.mainloop() |
How to choose a date on the widget?
Suppose I choose from 12-Apr to 16-Apr, then do the following image (the steps are marked with numbers):
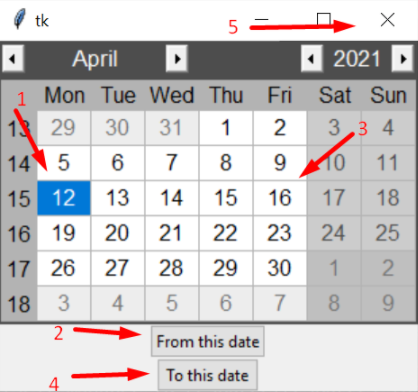
● Next, we will get all tasks via for loop and other infomation to create a table of report.
1 2 3 4 5 6 7 8 9 | print ("-------------------------------------------------------------------------------------------------") print ("||Module||PIC||Sub-leader||Task||Progress||Deadline||Delays(in days)||Issue/risk||Countermeasure||Note") time = redmine.time_entry.filter(user_id=userID, from_date=fromDate, to_date=toDate) for log in time: issue = redmine.issue.get(int(log.issue)) res = "#"+str(log.issue)+" - "+str(issue) res = res.replace('[', '[[') res = res.replace(']', ']]') print ("|"+module+"|"+pic+"|"+sublead+"|"+res+"|"+progress+"|"+deadline+"|"+delay+"|-|-|-|") |
● After completing the above steps, the result now is:

● Finally, I go to Confluence and paste the result to a report page.

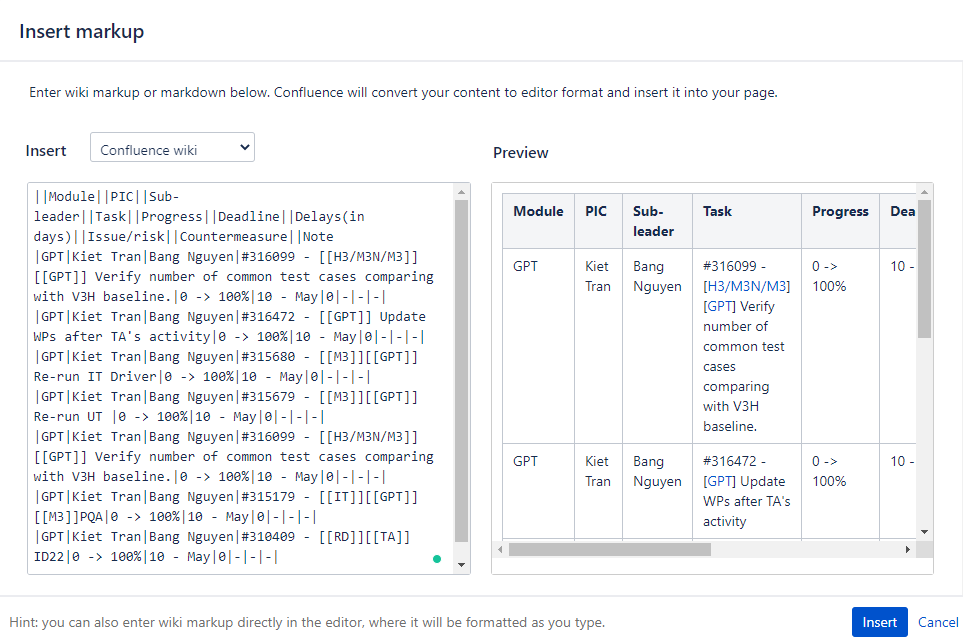

Here is full of source code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | import tkinter as tk from tkinter import ttk from tkcalendar import Calendar, DateEntry from redminelib import Redmine # ------- Modify here ------- # keyRedmine="5b0fd80da4413c8d7f33dec58942b96346dc9f51" userID="5158" module = "GPT" pic = "Kiet Tran" sublead = "Bang Nguyen" progress = "0 -> 100%" deadline = "10 - May" delay = "0" fromDate = "" toDate = "" # --------------------------- # root = tk.Tk() def Get_from_date(): global fromDate fromDate = str(cal.selection_get()) def Get_to_date(): global toDate toDate = str(cal.selection_get()) cal = Calendar(root,font="Arial 12", selectmode='day',cursor="hand2") cal.pack(fill="both", expand=True) ttk.Button(root, text="From this date", command=Get_from_date).pack() ttk.Button(root, text="To this date", command=Get_to_date).pack() root.mainloop() redmine = Redmine('https://socrm.dgn.renesas.com', key=keyRedmine,requests={'verify': False}) print ("-------------------------------------------------------------------------------------------------") print ("||Module||PIC||Sub-leader||Task||Progress||Deadline||Delays(in days)||Issue/risk||Countermeasure||Note") time = redmine.time_entry.filter(user_id=userID, from_date=fromDate, to_date=toDate) for log in time: issue = redmine.issue.get(int(log.issue)) res = "#"+str(log.issue)+" - "+str(issue) res = res.replace('[', '[[') res = res.replace(']', ']]') print ("|"+module+"|"+pic+"|"+sublead+"|"+res+"|"+progress+"|"+deadline+"|"+delay+"|-|-|-|") |