In this articale, I will use Python and JIRA library to log time into a task. Jira is an application that used to tracking and management a project, issue, and bug, developed to make this process easier for any organization.
Normally, when I want to log time in a day, I have to navigate to http://jira.(hidden-name).com.vn/, find the task I have worked in that day, fill time and description what I have worked.
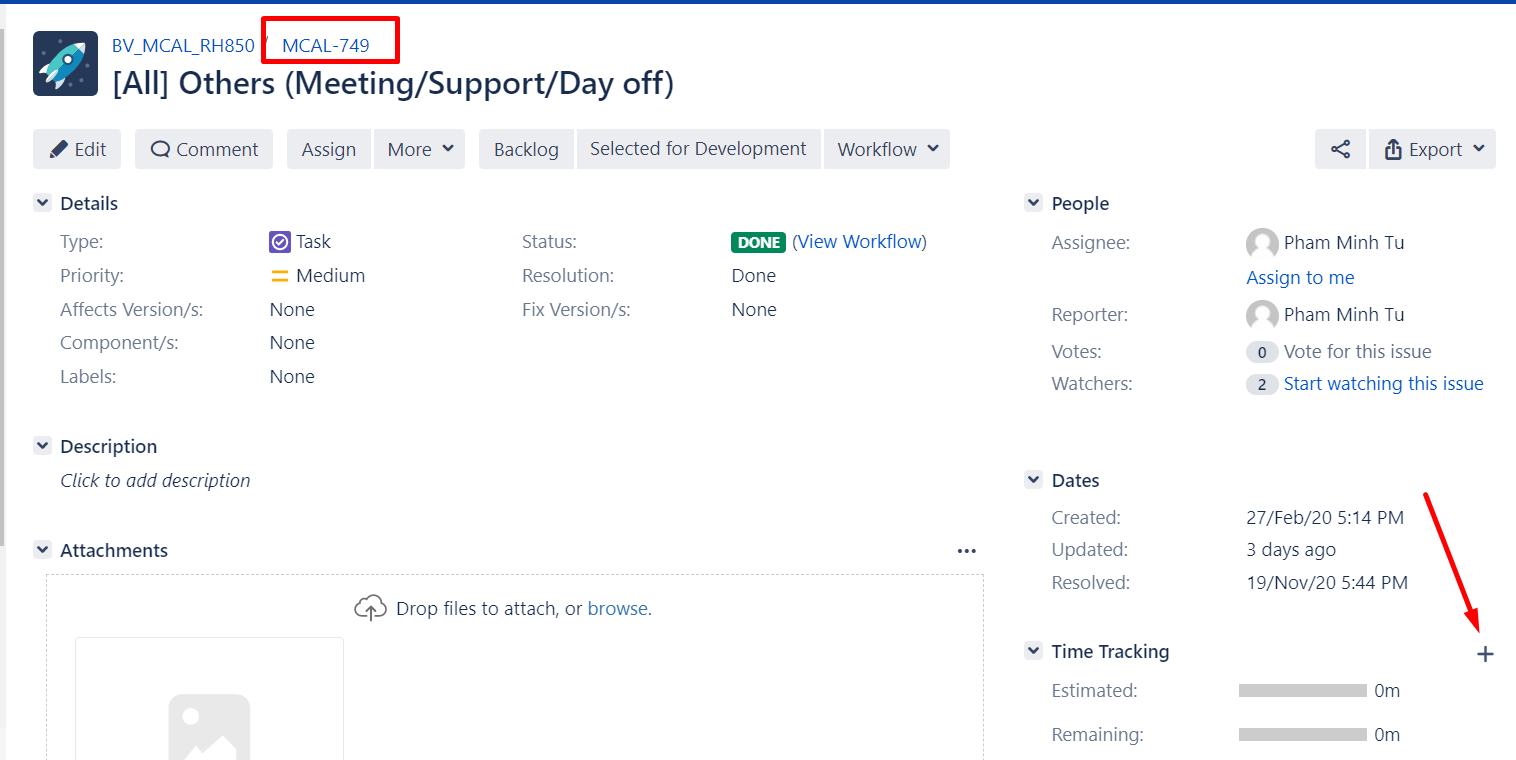
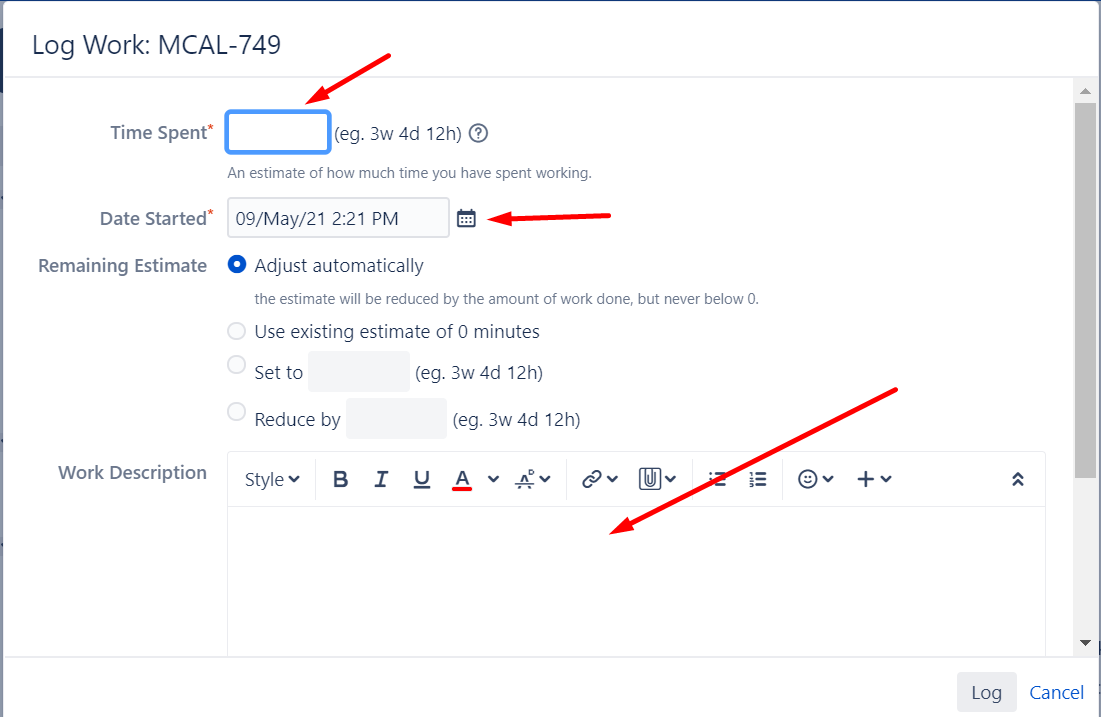
This will take time to do and I feel uncomfortable when doing so many steps. With this tool, it will listed all tasks that assigned to me. I just choose the task and log time.
Demo:
● Run command: python logworkJira.py. With my account, currently I am handling 2 tasks.
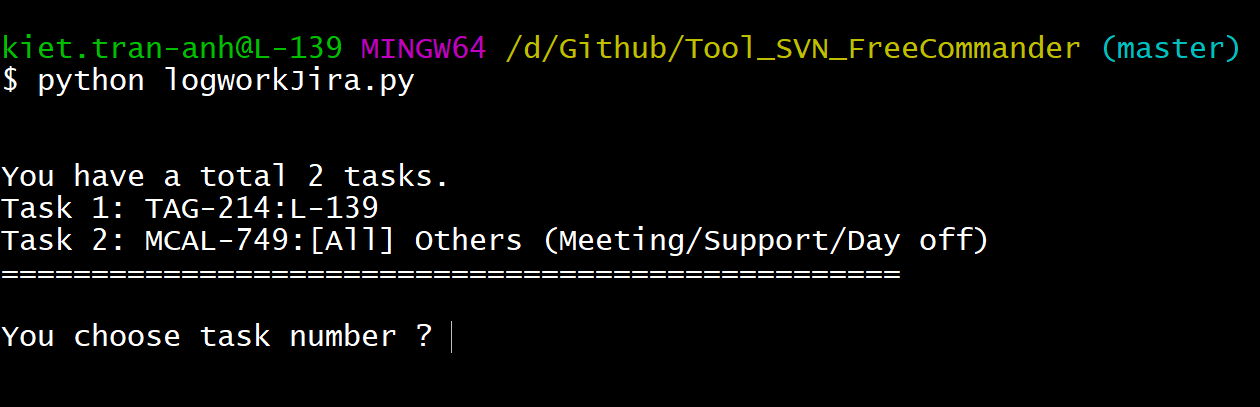
● The tool will ask me which task I want to choose to log work. For example, I choose the task number 2.
● Then, I will type the time I spent to do this task (2h) and my description (test log) and press Enter. The tool will ask me which date I want to choose to log work.
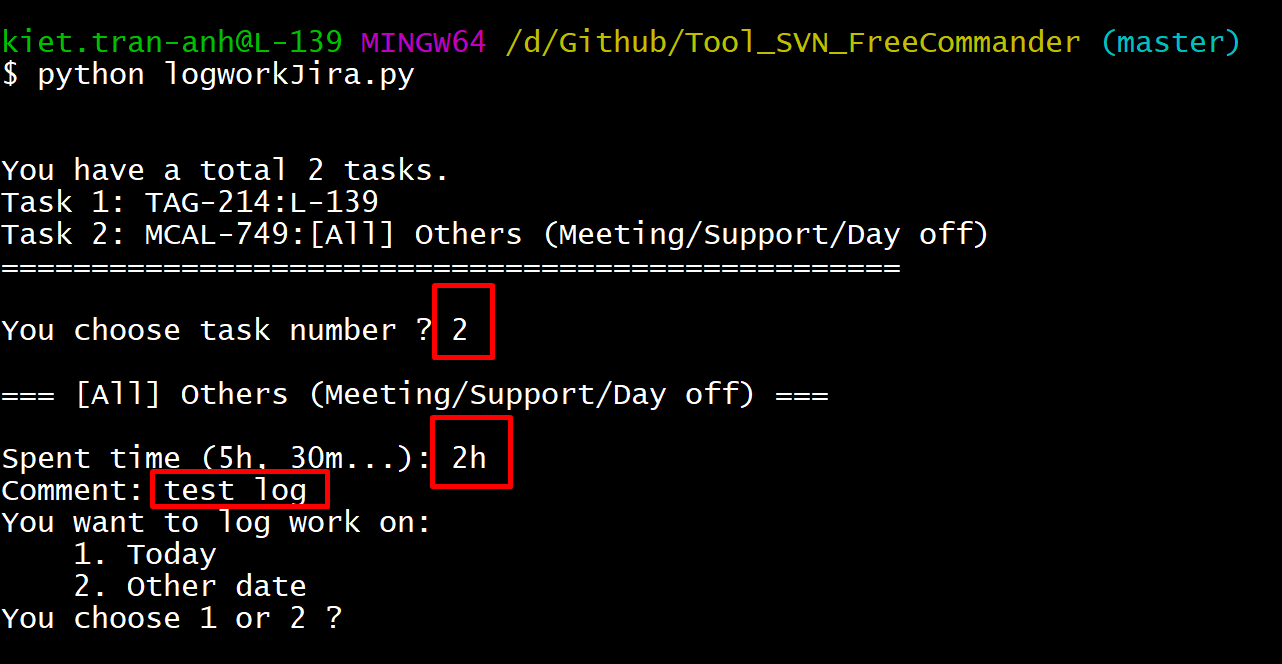
● I want to log work today, I will choose 1 and type Y. Then data will be sent to website of Jira.
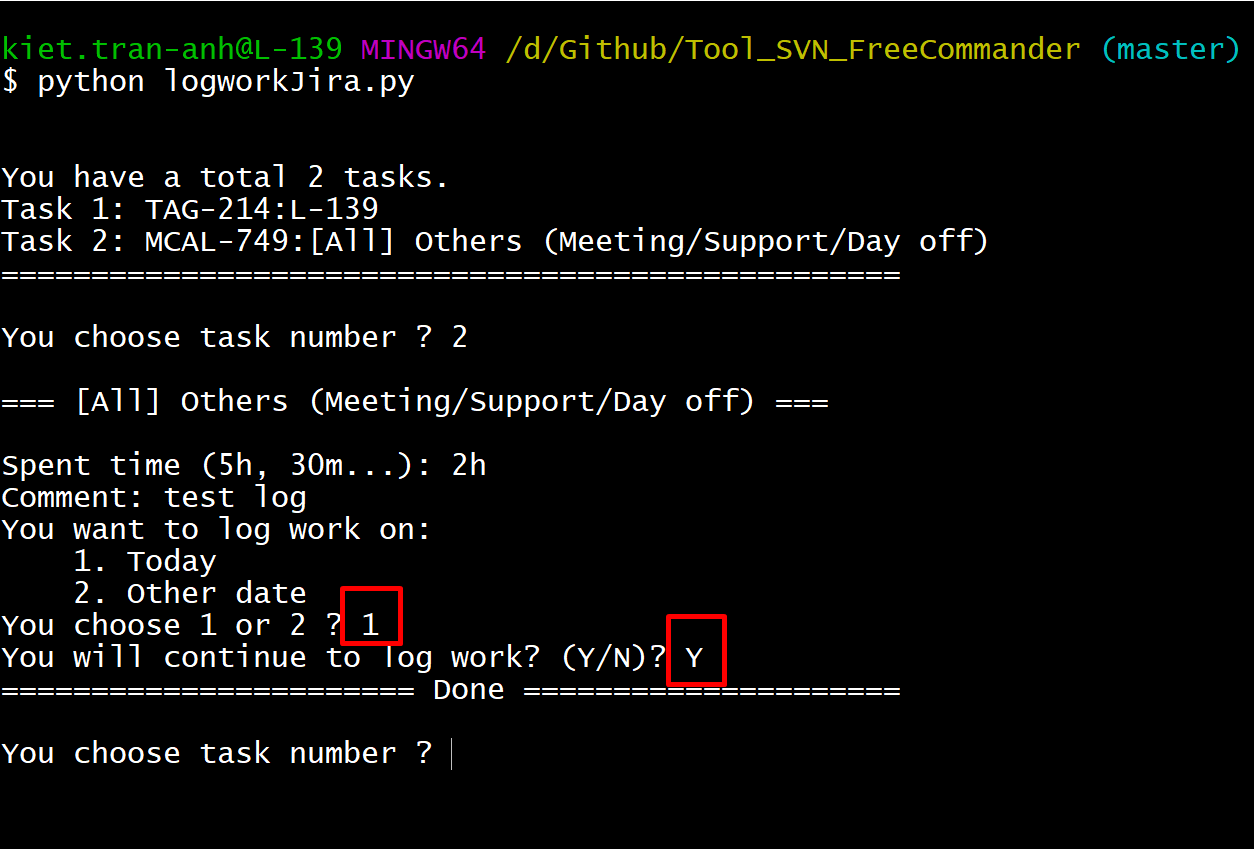
● I have to navigate to website of Jira and check the time log is correct or not.
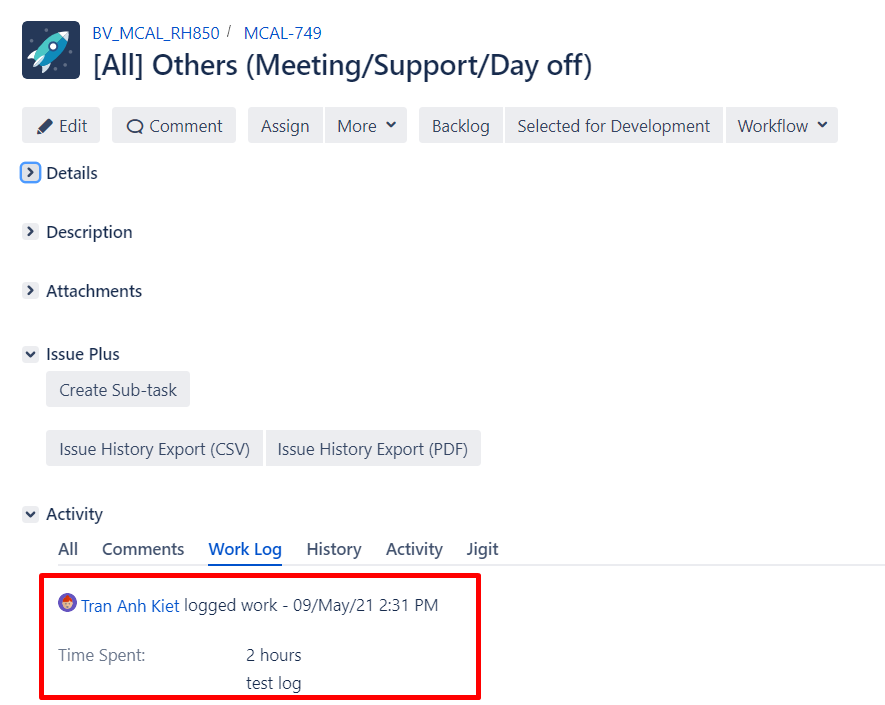
● This took can log time in any date. If I want to log to other date, I will choose 2 and fill the date format accordingly.
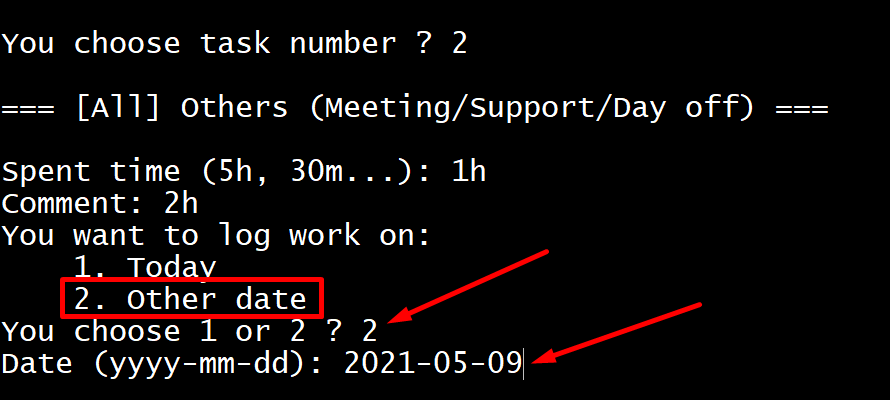
Now we will reseach how this tool work.
● Install JIRA library by using command: pip install JIRA.
1 | from jira import JIRA |
● Then, we will edit the link of JIRA website, username and password. Line 4 is used to log in to JIRA website.
1 2 3 4 | options = {"server": "https://jira.(hidden_name).com.vn"} user = "kiet.tran-anh" #your username log in JIRA pw = "xxxxxxxx" #your password log in JIRA jira = JIRA(options, basic_auth=(user,pw)) |
● Next, I will search all tasks that assigned to me and status is not done, then display the information on console output.
1 2 3 4 5 6 7 | issues = jira.search_issues("assignee=currentUser() and status!=done") print ("\n") print ("You have a total "+str(len(issues))+" tasks.") for i in range(0, len(issues)): pos = i print ("Task "+str(pos+1)+": "+ str(issues[i])+ ":"+str(issues[i].fields.summary)) print ("==================================================") |
● In this step, I will choose the task I want to log work. Then I will type the time, description and choose the date
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | value = input("\nYou choose task number ? ") ID_JIRA = issues[int(value)-1] print ("\n=== "+issues[int(value)-1].fields.summary+" ===\n") time = input("Spent time (5h, 30m...): ") com = input("Comment: ") print ("You want to log work on: ") print (" 1. Today") print (" 2. Other date") choice = input("You choose 1 or 2 ? ") if int(choice) == 2: date = input("Date (yyyy-mm-dd): ") date += "T17:20:00.000+0700" else: date = None |
● Finally, I will type "Y" to send data to JIRA website. If we type wrong time and comment, we will type "N" to do again.
1 2 3 4 5 6 7 | if yn == "Y": jira.add_worklog(ID_JIRA, timeSpent=time, comment=com, started=date) print ("======================= Done =====================") elif yn == "N": print ("===================== Canceled ===================") else: print ("===================== Try again ==================") |
Here is full of source code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | from jira import JIRA options = {"server": "https://jira.(hidden_name).com.vn"} user = "kiet.tran-anh" #your username log in JIRA pw = "xxxxxxxx" #your password log in JIRA jira = JIRA(options, basic_auth=(user,pw)) issues = jira.search_issues("assignee=currentUser() and status!=done") print ("\n") print ("You have a total "+str(len(issues))+" tasks.") for i in range(0, len(issues)): pos = i print ("Task "+str(pos+1)+": "+ str(issues[i])+ ":"+str(issues[i].fields.summary)) print ("==================================================") while 1: value = input("\nYou choose task number ? ") ID_JIRA = issues[int(value)-1] print ("\n=== "+issues[int(value)-1].fields.summary+" ===\n") time = input("Spent time (5h, 30m...): ") com = input("Comment: ") print ("You want to log work on: ") print (" 1. Today") print (" 2. Other date") choice = input("You choose 1 or 2 ? ") if int(choice) == 2: date = input("Date (yyyy-mm-dd): ") date += "T17:20:00.000+0700" else: date = None yn = input("You will continue to log work? (Y/N)? ") if yn == "Y": jira.add_worklog(ID_JIRA, timeSpent=time, comment=com, started=date) print ("======================= Done =====================") elif yn == "N": print ("===================== Canceled ===================") else: print ("===================== Try again ==================") |